はじめに
前回は JSX を使用せず Web 画面を作成しました。
今回は JSX を使用して Web 画面を作成する方法について説明します。
また、参考にした書籍は 「React ハンズオンラーニング」という本です。
これに沿って React の使用方法を学んでいこうと思います。
前提条件
前提条件は以下の通りです。
- Windows11
- React がインストールされている
- react-create-app を使用してパッケージが作成されている
プログラム作成
早速、プログラムを作成していきます。
今回も App.js にプログラムを記述していきます。
import React from "react";
function App() {
const data = [
{
name: "Baked Salmon",
ingredients: [
{ name: "Salmon", amount: 1, measurement: "l lb" },
{ name: "Pine Nuts", amount: 1, measurement: "cup" },
{ name: "Butter Lettuce", amount: 2, measurement: "cups" },
{ name: "Yellow Squash", amount: 1, measurement: "med" },
{ name: "Olive Oil", amount: 0.5, measurement: "cup" },
{ name: "Garlic", amount: 3, measurement: "cloves" },
],
steps: [
"Preheat the oven to 350 degrees.",
"Spread the olive oil around a glass baking dish.",
"Add the salmon, garlic, and pine nuts to the dish.",
"Bake for 15 minutes.",
"Add the yellow squash and put back in the oven for 30 mins.",
"Remove from oven and let cool for 15 minutes. Add the lettuce and serve.",
],
},
{
name: "Fish Tacos",
ingredients: [
{ name: "Whitefish", amount: 1, measurement: "l lb" },
{ name: "Cheese", amount: 1, measurement: "cup" },
{ name: "Iceberg Lettuce", amount: 2, measurement: "cups" },
{ name: "Tomatoes", amount: 2, measurement: "large" },
{ name: "Tortillas", amount: 3, measurement: "med" },
],
steps: [
"Cook the fish on the grill until hot.",
"Place the fish on the 3 tortillas.",
"Top them with lettuce, tomatoes, and cheese",
],
},
];
return (
<>
{data.map((d, i) => (
<section id={i}>
<h1>{d.name}</h1>
{d.steps.map((s)=>(
<li>{s}</li>
))}
</section>
))}
</>
)
}
export default App;
npm start でローカルサーバーを起動し、http://localhost:3000/ へアクセスします。
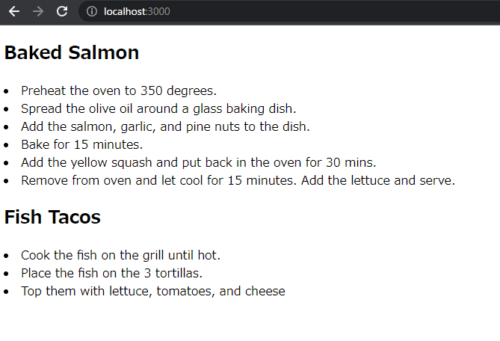
return 文以降の説明をします。
return (
<>
こちらの <></> は、最も外側に配置します。大外で囲わないとエラーが出ます。
{data.map((d, i) => (
data 変数を map で一つずつ展開していきます。python の for ループのイメージです。
d は data 変数、i は インデックスです。
<section id={i}>
<section> タグです。map 関数内のタグは id を指定しないとワーニングが出ます。
<h1>{d.name}</h1>
<h1> タグで、data 変数の name を表示します。
{d.steps.map((s)=>(
<li>{s}</li>
))}
data 変数の step 部分を map 関数で更にループさせます。
<li> タグで step をリスト表示します。
おわりに
今回は JSX を使用して画面を作成する方法について説明しました。
JSX は React を使用するうえでは頻繁に登場します。
次回は、口コミ等で使用される評価の星のアニメーションを取り扱います。
コメント